FAN CONTROL:
Well after some thought and plenty of carelessness to read the fuckin' manual ..
Thus 'mishappens' ...
I've ended up with this solution:
Voltage control of the fans .
Those Sunons have an operating range of 6-13.8 VDC .
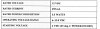
(Big "Thank you" goes to Guod ,for supplying with the datasheet.Something I neglected to find & read.. )
So ,I'm going to use this circuit :
The formula to set the output voltage is this :
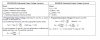
R1 is going to be
1K ( 1000 ohms )
R2 is not going to be a pot ....I have handy a spare and brand new ,
1x12 rotary switch ,with a nice "clic-clic-clic-clic-".. 'movement/action' ...
It is going to be placed the other side of the round monitor ...
One knob for cooling control ,one knob for light output control ..
I do not want a pot ,as it will go from 0 to 10 K ,for example ...
This means an output voltage of ~1.2 to ~13.5 ...
I want the lowest setting to be around 7 VDC ..Not 1.2 VDC ...
I want to ensure that fans will start to roll ,once the timer switches on the fixture ...
Even with plenty of dust and debris ,sitted on their 'wings/rotors' ...
Making them heavier,needy of more power (=>voltage) to start rolling ..
So a rotary switch ...
With min setting R2= 4700 K
and advancing for 11x settings,each setting + 470 Ohms
total = 4700+ {470*11}=9870
V out max = 1.23* ( 1+ { 9870/1000} ) =>
V out max=13.37 =~ 13.4 VDC
Vout min= 1.23* ( 1+ { 4700/1000} )=>
V out min=7.011 =~ 7 VDC
Settings " 9" & "10 " are ~11.6 & ~12.2 VDC respectively ..
The "
Nominal Operation " ...
Settings '11' & '12 ' are the '
Soft Overdrive Operation' ..
( ~12.8 & ~13.4 respectively )
Settings '1' to '4' are the '
Silent Operation'
( ~7 to ~8.75 VDC )
Settings '5' to '8' are the '
Eco Operation'
( ~9.3 to ~11 VDC )
In few hours time ,an update on that ...